In Flutter, you can use the Table widget to create a table that organizes its children in a two-dimensional arrangement. Here’s an example of using the Table widget:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Table Example'),
),
body: TableExample(),
),
);
}
}
class TableExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Table(
border: TableBorder.all(),
columnWidths: const <int, TableColumnWidth>{
0: IntrinsicColumnWidth(),
1: FlexColumnWidth(),
2: FixedColumnWidth(64),
},
defaultVerticalAlignment: TableCellVerticalAlignment.middle,
children: [
TableRow(
children: [
Container(
color: Colors.green,
height: 48,
child: Center(child: Text('Header 1')),
),
Container(
color: Colors.green,
height: 48,
child: Center(child: Text('Header 2')),
),
Container(
color: Colors.green,
height: 48,
child: Center(child: Text('Header 3')),
),
],
),
TableRow(
children: [
Container(
height: 48,
child: Center(child: Text('Row 1, Cell 1')),
),
Container(
height: 48,
child: Center(child: Text('Row 1, Cell 2')),
),
Container(
height: 48,
child: Center(child: Text('Row 1, Cell 3')),
),
],
),
TableRow(
children: [
Container(
height: 48,
child: Center(child: Text('Row 2, Cell 1')),
),
Container(
height: 48,
child: Center(child: Text('Row 2, Cell 2')),
),
Container(
height: 48,
child: Center(child: Text('Row 2, Cell 3')),
),
],
),
],
);
}
}
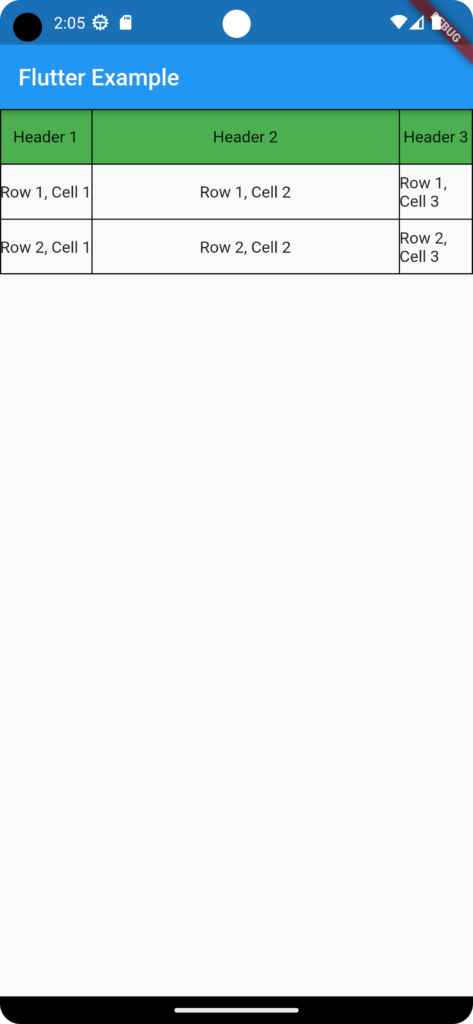