Rotate widget in flutter | Rotate Container in flutter | Rotate Text in flutter | Rotate Icon in flutter
In Flutter, you can rotate a widget, container, or text using the Transform widget. The Transform widget allows you to apply various transformations, including rotation of widget, adjusting the angle of the widget. Here are examples of rotating a widget, a container, and text in Flutter:
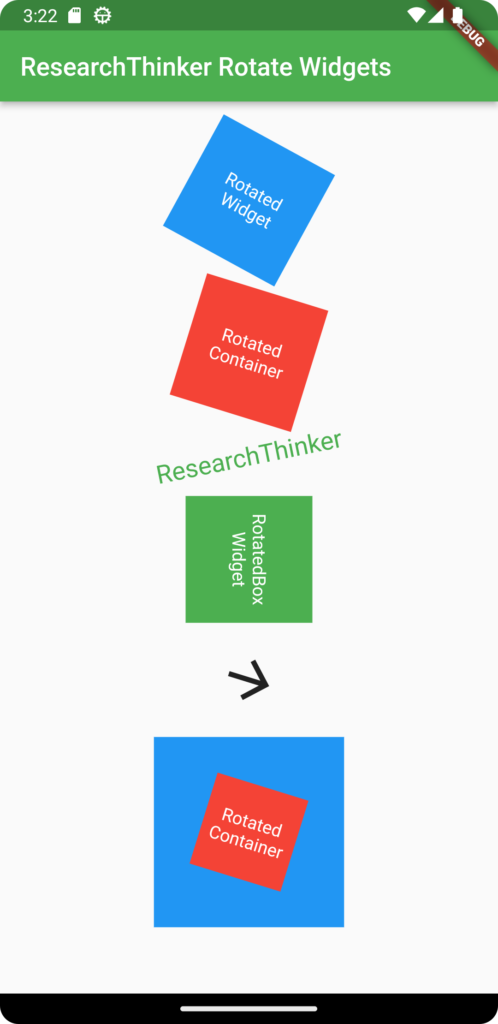
how to rotate a widget in flutter ?
Center(
child: Transform.rotate(
angle: 0.5, // Specify the rotation angle in radians
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(
child: Text(
'Rotated Widget',
style: TextStyle(color: Colors.white),
),
),
),
),
),
how to rotate container in flutter ?
Center(
child: Transform.rotate(
angle: 0.3, // Specify the rotation angle in radians
child: Container(
width: 100,
height: 100,
color: Colors.red,
child: Center(
child: Text(
'Rotated Container',
style: TextStyle(color: Colors.white),
),
),
),
),
),
how to rotate text in flutter ?
Center(
child: Transform.rotate(
angle: -0.2, // Specify the rotation angle in radians
child: Text(
'ResearchThinker',
style: TextStyle(fontSize: 20),
),
),
),
Full code of all widgets:-
import 'package:flutter/material.dart';
class RotateWidgets extends StatefulWidget {
const RotateWidgets({Key? key}) : super(key: key);
@override
State<RotateWidgets> createState() => _RotateWidgetsState();
}
class _RotateWidgetsState extends State<RotateWidgets> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('ResearchThinker Rotate Widgets'),
),
body: SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.all(28.0),
child: Column(children: [
//Rotated Widget
Center(
child: Transform.rotate(
angle: 0.5, // Specify the rotation angle in radians
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(
child: Text(
'Rotated \n Widget',
style: TextStyle(color: Colors.white),
textAlign: TextAlign.center,
),
),
),
),
),
commonGap(),
// Rotated Container
Center(
child: Transform.rotate(
angle: 0.3, // Specify the rotation angle in radians
child: Container(
width: 100,
height: 100,
color: Colors.red,
child: Center(
child: Text(
'Rotated Container',
style: TextStyle(
color: Colors.white,
),
textAlign: TextAlign.center,
),
),
),
),
),
commonGap(),
//Rotate Text
Center(
child: Transform.rotate(
angle: -0.2, // Specify the rotation angle in radians
child: Text(
'ResearchThinker',
style: TextStyle(fontSize: 20, color: Colors.green),
),
),
),
commonGap(),
//RotatedBox Widget
Center(
child: RotatedBox(
quarterTurns: 1, // Number of clockwise quarter turns
child: Container(
width: 100,
height: 100,
color: Colors.green,
child: Center(
child: Text(
'RotatedBox Widget',
style: TextStyle(color: Colors.white),
textAlign: TextAlign.center,
),
),
),
),
),
commonGap(),
//Rotate Icon
Center(
child: Transform.rotate(
angle: 0.3, // Specify the rotation angle in radians
child: Icon(
Icons.arrow_forward,
size: 50,
),
),
),
commonGap(),
//Containers overlap through stack and rotate through Transform
Center(
child: Stack(
alignment: Alignment.center,
children: [
// Bottom Container
Container(
width: 150,
height: 150,
color: Colors.blue,
),
// Top Container with Rotation
Transform.rotate(
angle: 0.3, // Specify the rotation angle in radians
child: Container(
width: 75,
height: 75,
color: Colors.red,
child: Center(
child: Text(
'Rotated Container',
style: TextStyle(color: Colors.white),
textAlign: TextAlign.center,
),
),
),
),
],
),
),
]),
),
));
}
commonGap() {
return SizedBox(
height: 20,
);
}
}
Conclusion: In Flutter, through Transform widget we can rotate any widget like container, text, icon, etc. In the above example through transform widget we have rotated all the widgets to specific, similarly you can use in your application also.