Alert Dialog box not visible
Flutter Tutorial:
Install Flutter in Win/Linux/Mac
Flutter Widgets:
Bottom Navigation Bar in Flutter
Auto Close Keyboard in Flutter
Screen size handling in Flutter
Flutter REST API
Flutter Advance
Wrap vs Builder vs OverBarFlow
Circular progress contain Icon
Flutter State management Comparison
Flutter Database
Flutter Token Expired Handling
Flutter Provider
Flutter GetX
Flutter with Native
Flutter Tips
Interview Questions
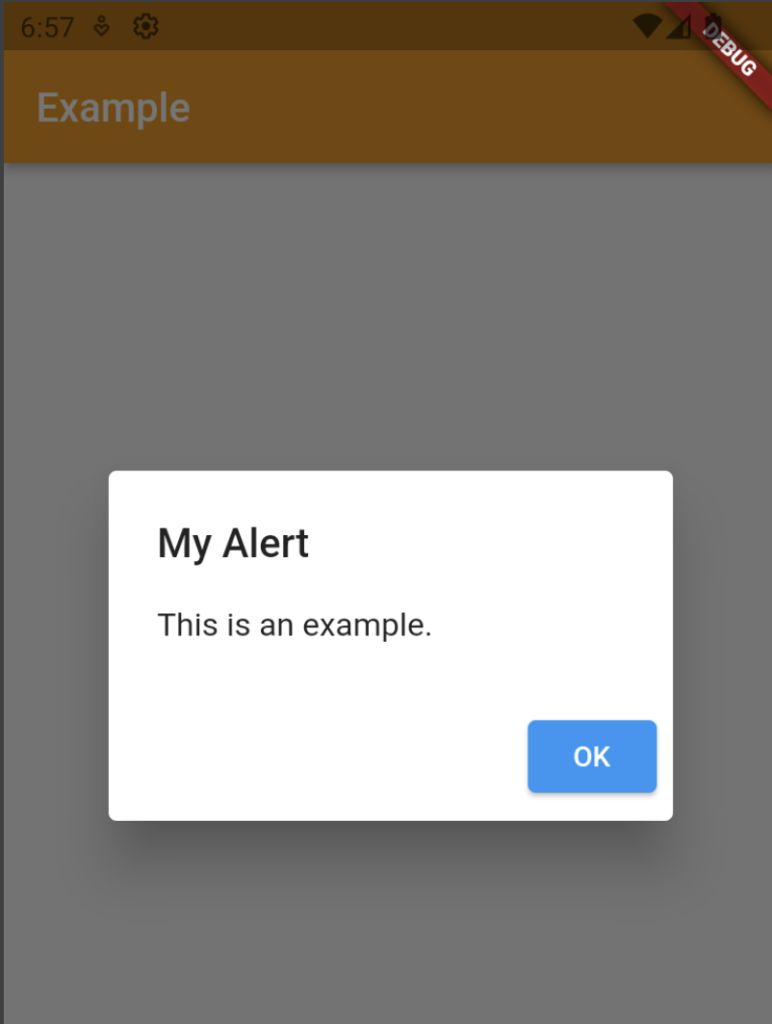
If your Alert Dialog is not visible or not open, in your Flutter app, one of the most common reason could be that you are not passing the correct BuildContext. BuildContext play a significant role, in Flutter
Here in this case, we are calling function without passing context as a parameter, so this function which contain AlertDialog box will never call or never open.
// Incorrect Example
void showAlertDialog() {
AlertDialog(
title: Text('My Alert'),
content: Text('This is an example.'),
actions: [
ElevatedButton(
onPressed: () {
Navigator.of(context).pop(); // This won't work without the correct context.
},
child: Text('OK'),
),
],
);
}
Above example is incorrect , if you try to call showAlertDialog without passing the context , the dialog won’t be open because it is not associated with any part of the widget tree, and it may not appear as expected.
To resolve this issue, make sure you pass the correct BuildContext when showing the dialog
Here in this case, showAlertDialog pass context as a parameter, so alert dialog box open smoothly
// Correct Example
void showAlertDialog(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('My Alert'),
content: Text('This is an example.'),
actions: [
//This is an elevated button, when user clicks it // will go to previous page due to // //Navigator.of(context).pop().
ElevatedButton(
onPressed: () {
Navigator.of(context).pop(); //
},
child: Text('OK'),
),
],
);
},
);
}
Second Issue when Alert Dialog box not Open
Sometimes the alert dialog box does not open even if we are using the correct syntax, then you need to check the size of the alert dialog box, sometimes due to the size issue, it blocks the screen and the alert dialog box does not open smoothly
To tackle this issue adjust height and width
void showAlertDialog(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('My Alert'),
content: Container(
height:300, // use media query instead of 300
width:300,// use media query instead of 300
child: AnyWidget(),),
actions: [
ElevatedButton(
onPressed: () {
Navigator.of(context).pop(); // This will work with the correct context.
},
child: Text('OK'),
),
],
);
},
);
}
Full Code of Alert Dialog box:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyAppExampleAlertDialog(),
);
}
}
class MyAppExampleAlertDialog extends StatelessWidget {
@override
Widget build(BuildContext context) {
final dynamicColor = Colors.orange;
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Example'),
backgroundColor: dynamicColor,
),
body: Center(
child: Container(
color: dynamicColor,
child: InkWell(
// In this case we call show alert dialog box and it will work because we pass context , otherwise it will not work
onTap:(){
showAlertDialog(context);
},
child: Text(
'Hello, Flutter!',
style: TextStyle(color: Colors.white),
),
),
),
),
),
);
}
void showAlertDialog(BuildContext context) {
showDialog(
// here in this context belong to MyAppExampleAlertDialog so it will work smoothly
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('My Alert'),
content: Text('This is an example.'),
actions: [
ElevatedButton(
onPressed: () {
Navigator.of(context).pop(); // This will work with the correct context.
},
child: Text('OK'),
),
],
);
},
);
}
}
Conclusion: The most common mistakes that create blocker while integrating alert dialog boxes in Flutter are missing context or size issues, based on above example hope you will not face any issue while integrating alert dialog boxes or any type of dialog box.