How to implement Code Editor in Flutter
Flutter Tutorial
Install Flutter in Win/Linux/Mac
Flutter Widgets:
Bottom Navigation Bar in Flutter
Auto Close Keyboard in Flutter
Screen size handling in Flutter
Flutter REST API
Flutter Advance
Wrap vs Builder vs OverBarFlow
Circular progress contain Icon
Flutter State management Comparison
Flutter Database
Flutter Token Expired Handling
Flutter Provider
Flutter GetX
Flutter with Native
Flutter Tips
Interview Questions
In Flutter, a code editor refers to a text editor or an IDE, where developers write, edit, and manage their Flutter code. A code editor provides a user-friendly interface with features such as syntax highlighting
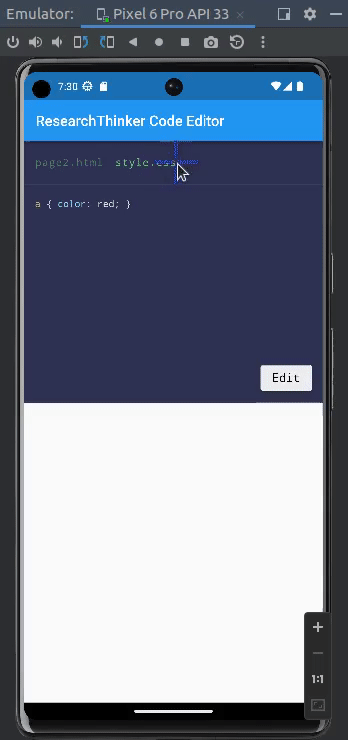
In this we use code_editor package
code_editor: # use latest version
In thi package you view and edit , HTML, Dart and Js code
import 'package:flutter/material.dart';
import 'package:rttutorials/code_editor.dart';
import 'package:code_editor/code_editor.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'ResearchThinker',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: CodeEditorFile(),//ResearchThinkerExample(),
);
}
}
class CodeEditorFile extends StatelessWidget {
const CodeEditorFile({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
// example of a easier way to write code instead of writing it in a single string
List<String> contentOfPage1 = [
"<!DOCTYPE html>",
"<html lang='fr'>",
"\t<body>",
"\t\t<a href='page2.html'>go to page 2</a>",
"\t</body>",
"</html>",
];
// The files displayed in the navigation bar of the editor.
// You are not limited.
// By default, [name] = "file.${language ?? 'txt'}", [language] = "text" and [code] = "",
List<FileEditor> files = [
FileEditor(
name: "page2.html",
language: "html",
code: "<a href='page1.html'>go back</a>",
),
FileEditor(
name: "style.css",
language: "css",
code: "a { color: red; }",
),
];
// The model used by the CodeEditor widget, you need it in order to control it.
// But, since 1.0.0, the model is not required inside the CodeEditor Widget.
EditorModel model = EditorModel(
files: files, // the files created above
// you can customize the editor as you want
styleOptions: EditorModelStyleOptions(
fontSize: 13,
),
);
// A custom TextEditingController.
final myController = TextEditingController(text: 'hello!');
return Scaffold(
appBar: AppBar(title: const Text("ResearchThinker Code Editor")),
body: SingleChildScrollView(
// /!\ important because of the telephone keypad which causes a "RenderFlex overflowed by x pixels on the bottom" error
// display the CodeEditor widget
child: CodeEditor(
model: model, // the model created above, not required since 1.0.0
edit: true, // can edit the files? by default true
onSubmit: (String? language, String? value) => print("yo"),
disableNavigationbar:
false, // hide the navigation bar ? by default false
textEditingController:
myController, // Provide an optional, custom TextEditingController.
),
),
);
}
}