Flutter Tutorial:
Flutter Widgets:
Flutter Advance
Flutter REST API
Advanced Concepts
Wrap vs Builder vs OverBarFlow
Circular progress contain Icon
Flutter State management Comparison
Flutter Database
Flutter Token Expired Handling
Flutter Provider
Flutter GetX
Flutter with Native
Flutter Tips:
Interview Questions
Flutter 100 Interview Questions
Custom widget in flutter
Using custom widgets is one of the best practices, it not only reduces code but also improves efficiency. In Flutter applications, we generally implement custom widgets where we need common functionality such as entering text fields (email, password, mobile number, etc.). The most common example of a custom widget requiring a text field
To create a custom text field in Flutter that allows users to modify the text field according to their needs, below code clearly explain
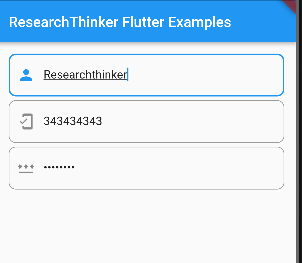
CustomTextField widget Example
import 'package:flutter/material.dart';
import 'package:rttutorials/custom_text_field.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'ResearchThinker',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyAppCardExample(),
);
}
}
class MyAppCardExample extends StatelessWidget {
const MyAppCardExample({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('ResearchThinker Flutter Examples'),
),
body: SingleChildScrollView(
child: Container(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
//Here we integrate customTextField instead of normal Textfield and we can use this CustomTextField multiple times as per our requirements
CustomTextField(
controller: TextEditingController(),
hintText: 'Enter your name',
icon: Icons.person,
),
const SizedBox(
height: 5,
),
CustomTextField(
controller: TextEditingController(),
hintText: 'Enter Mobile Number',
icon: Icons.mobile_friendly,
),
const SizedBox(
height: 5,
),
CustomTextField(
controller: TextEditingController(),
hintText: 'Enter Password',
icon: Icons.password,
obscureText: true,
)
],
),
),
),
),
);
}
}
// you can create different dart file for below code, in this example we //create file name custom_text_field.dart
import 'package:flutter/material.dart';
//In this way you can create custom text field, this custom widget is used for text related services
class CustomTextField extends StatefulWidget {
final TextEditingController controller;
final String hintText;
final IconData icon;
final bool obscureText;
CustomTextField({
required this.controller, // required mean compulsory to pass parameters
required this.hintText,
required this.icon,
this.obscureText = false,
});
@override
_CustomTextFieldState createState() => _CustomTextFieldState();
}
class _CustomTextFieldState extends State<CustomTextField> {
@override
Widget build(BuildContext context) {
//Note: If you want to add more parameters, then you have decalre also like widget.obscureText
return TextFormField(
controller: widget.controller,
obscureText: widget.obscureText,
decoration: InputDecoration(
hintText: widget.hintText,
prefixIcon: Icon(widget.icon),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(10.0),
),
),
);
}
}
In this example, the CustomTextField widget takes several parameters:
- controller: The TextEditingController to manage the text field’s value, which behave similar like normal TextFormField.
- hintText: The placeholder text to display inside the text field, this one also behave similar like TextFormField.
- icon: The icon to show as a prefix inside the text field.(you can also add Sufix Icon in this)
- obscureText: A flag indicating whether the text should be visible or not (e.g., for password fields).
Remaning parameters are same like TextFormField
You can use this CustomTextField widget in your Flutter application , the syntax of declaring customTextField is :-
CustomTextField(
controller: TextEditingController(),
hintText: 'Enter your name',
icon: Icons.person,
)
If you face any issue, please comment below, we will reply soon