Flutter Tutorial
Install Flutter in Win/Linux/Mac
Flutter Widgets:
Bottom Navigation Bar in Flutter
Auto Close Keyboard in Flutter
Screen size handling in Flutter
Flutter REST API
Flutter Advance
Wrap vs Builder vs OverBarFlow
Circular progress contain Icon
Flutter State management Comparison
Flutter Database
Flutter Token Expired Handling
Flutter Provider
Flutter GetX
Flutter with Native
Flutter Tips
Interview Questions
What is dio in Flutter ?
Dio is a powerful HTTP client for Dart and Flutter that supports features like interceptors, FormData, cancellation, and many more, It is used for connectivity flutter UI with backends such as Nodejs.
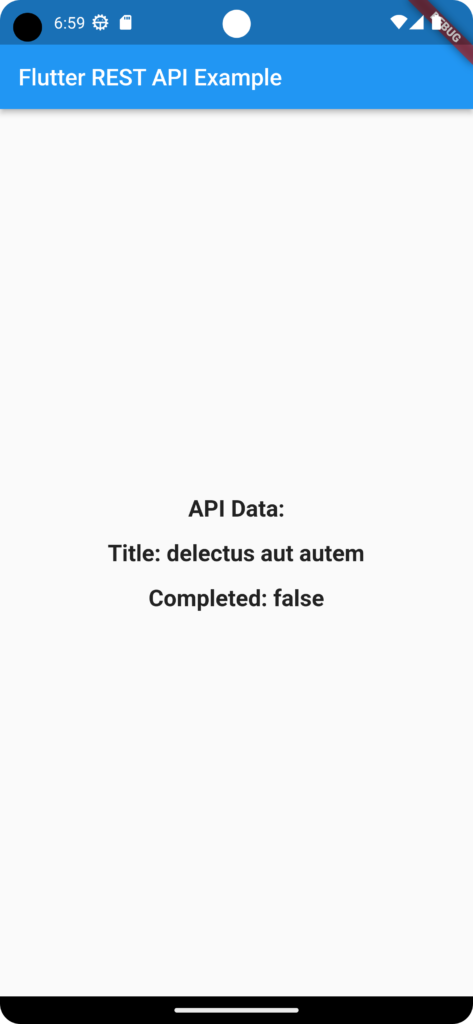
How to use Dio in a Flutter app ?
First add dio package in pubspec.yaml file
dependencies:
dio: # use latest version
After adding the dependency to the pubspec file, you can use the dio package to make API requests.
import 'package:flutter/material.dart';
import 'package:dio/dio.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Dio Flutter Example'),
),
body: ApiExample(), //Here we call APiExample
),
);
}
}
//This is API model which is used for retereive data in Object form from API
class Todo {
final int userId;
final int id;
final String title;
final bool completed;
Todo({
required this.userId,
required this.id,
required this.title,
required this.completed,
});
factory Todo.fromJson(Map<String, dynamic> json) {
return Todo(
userId: json['userId'],
id: json['id'],
title: json['title'],
completed: json['completed'],
);
}
}
class ApiExample extends StatefulWidget {
@override
_ApiExampleState createState() => _ApiExampleState();
}
class _ApiExampleState extends State<ApiExample> {
final String apiUrl = 'https://jsonplaceholder.typicode.com/todos/1'; //For testing purpose we use this url which provide Json data
late Todo todo;
@override
void initState() {
super.initState();
fetchData();
}
Future<void> fetchData() async {
try {
final response = await Dio().get(apiUrl);
if (response.statusCode == 200) {
// If the server returns a 200 OK response, parse the JSON
setState(() {
todo = Todo.fromJson(response.data);
});
} else {
// If the server did not return a 200 OK response,
// throw an exception.
throw Exception('Failed to load data');
}
} catch (error) {
print('Error: $error');
}
}
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('API Data:'),
SizedBox(height: 16),
//Here API data display
Text('Title: ${todo.title}'),
SizedBox(height: 16),
Text('Completed: ${todo.completed}'),
],
),
);
}
}
If you face any issue, please comment below, we will reply soon