const vs final in Flutter with example
Flutter Tutorial
Install Flutter in Win/Linux/Mac
Flutter Widgets:
Bottom Navigation Bar in Flutter
Auto Close Keyboard in Flutter
Screen size handling in Flutter
Flutter REST API
Flutter Advance
Wrap vs Builder vs OverBarFlow
Circular progress contain Icon
Flutter State management Comparison
Flutter Database
Flutter Token Expired Handling
Flutter Provider
Flutter GetX
Flutter with Native
Flutter Tips
Interview Questions
In flutter const and final are very commonly used and both are used for different purposes, const value are known at compile-time whereas , final value determined at runtime. Both const and final promote immutability, contributing to safer and more predictable code.
const | final |
Immutable, cannot be changed after creation. | Immutable, cannot be changed after initialization. |
Must be a compile-time constant value. | Can be initialized at runtime but cannot be reassigned. |
Commonly used for constant values like numbers, strings, and instances of user-defined classes with const constructors. | Commonly used for values that can be determined at runtime but won’t change thereafter. |
Must be initialized with a constant value at compile-time. | Must be initialized either at runtime or with a value that can be determined at runtime. |
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
//In this we use const and final in same
// Here const for compile-time constants
static const int compileTimeConstant = 42;
static const String compileTimeString = "Hello, const!";
// Here final for values determined at runtime
final int runtimeValue = DateTime.now().second;
final String runtimeString = "Hello, final!";
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('ResearchThinker: const vs final in Flutter'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
//when we run the program and refresh the values of runtime will change
Text('Compile-time constant: $compileTimeConstant'),
Text('Compile-time string: $compileTimeString'),
SizedBox(height: 20),
Text('Runtime value: $runtimeValue'),
Text('Runtime string: $runtimeString'),
],
),
),
),
);
}
}
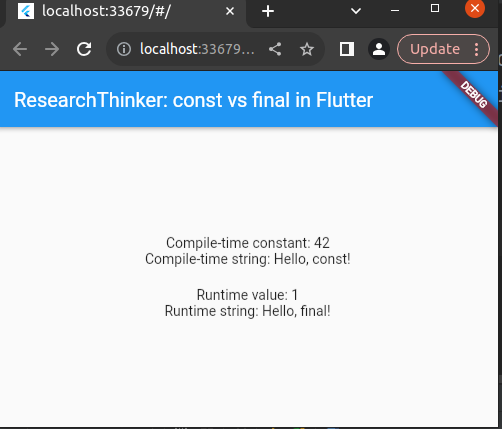
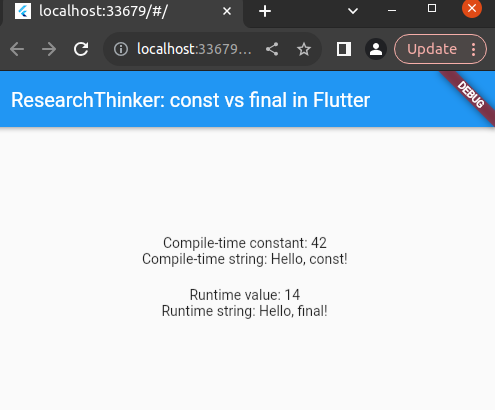
Here we execute this program and use web (chrome), so above screenshots related to this program clearly shows that when we refresh the runtime value change and compile value remain same.
compileTimeConstant and compileTimeString are declared with const because their values are known at compile time.
runtimeValue and runtimeString are declared with final because their values are determined at runtime.